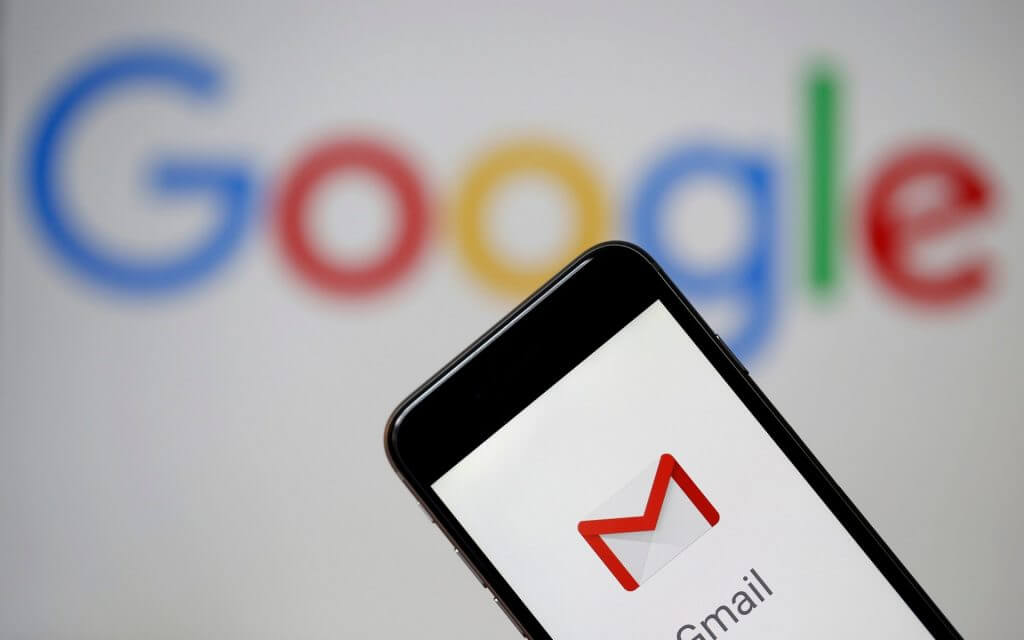
What is smtpLib
We can easily send email from python script using smtplib module, which defines an SMTP client session object that can be used to send mail to any Internet machine with an SMTP or ESMTP listener daemon.
Import smtplib module
Letโs create a simple script for the same by importing smtplib module.
import smtplib
from email.MIMEMultipart import MIMEMultipart
from email.MIMEText import MIMEText
Please use different methods if you are using different version of python. Eg: show below.
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
Prepare Email Configurations
You may add this items directly inside the script (hardcoded) or add as a separate configuration file outside.
email_from_addr = "myfromemail@gmail.com"
email_to_addr = "mytoemail@gmail.com"
email_smtp_server = "smtp.gmail.com"
email_smtp_port = "587"
email_user = "myfromemail"
email_password = "mypassword"
#Email Subject
email_subject = "Welcome to Python Email Test"
Prepare Email Body
This is not mandatory to test but adding a better way to handle multipart and rich email content.
# HTML + CSS for body
email_body = None
email_body_header = ' '
email_body_header = email_body_header + '<html><head></head><body>'
email_body_header = email_body_header + '<style type="text/css"></style>'
email_body_header = email_body_header + '<br><p>Hello Team,<br><br>This is a test email.<br>'
email_body_content = ' '
email_body_content = email_body_content + '<H1>This is main content area</h1>'
email_body_footer = ' '
email_body_footer = email_body_footer + '<br>Thank you'
email_body_footer = email_body_footer + '<br>Support Team<br>'
email_body = str(email_body_header) + str(email_body_content) + str(email_body_footer)
Prepare Email Content
Now, we have all details of email and letโs stitch all together.
message = MIMEMultipart('alternative')
message['From'] = email_from_addr
message['To'] = email_to_addr
message['Subject'] = email_subject
body = email_body
message.attach(MIMEText(body, 'html'))
# print (email_body)
Send Email
Now with prepared data, we will create a connection and send email over that.
server = smtplib.SMTP(email_smtp_server,int(email_smtp_port))
text = message.as_string()
server.starttls()
server.login(email_user, email_password)
server.sendmail(email_from_addr,email_to_addr,message.as_string())
server.quit()
Note : If you are using an open smtp connection (with whitelisted IP), you donโt need to do login as authentication will happen automatically (if you configured your SMTP server in such a way).
Check Email Received
You can access the full script from github โ python-email.py.
Refer SMTPLib documentation for more details.